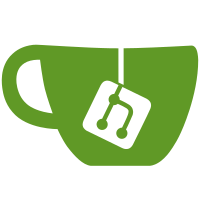
committed by
GitHub
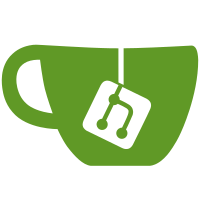
8 changed files with 175 additions and 32 deletions
@ -0,0 +1,109 @@ |
|||||
|
/*
|
||||
|
* vim:ts=4:sw=4:expandtab |
||||
|
* |
||||
|
* i3 - an improved dynamic tiling window manager |
||||
|
* © 2009 Michael Stapelberg and contributors (see also: LICENSE) |
||||
|
* |
||||
|
*/ |
||||
|
#include "dpi.h" |
||||
|
|
||||
|
#include <math.h> |
||||
|
#include <stdlib.h> |
||||
|
#include <stdio.h> |
||||
|
#include <xcb/xcb_xrm.h> |
||||
|
#include "xcb.h" |
||||
|
#include "i3lock.h" |
||||
|
|
||||
|
extern bool debug_mode; |
||||
|
|
||||
|
static long dpi; |
||||
|
|
||||
|
extern xcb_screen_t *screen; |
||||
|
|
||||
|
static long init_dpi_fallback(void) { |
||||
|
return (double)screen->height_in_pixels * 25.4 / (double)screen->height_in_millimeters; |
||||
|
} |
||||
|
|
||||
|
/*
|
||||
|
* Initialize the DPI setting. |
||||
|
* This will use the 'Xft.dpi' X resource if available and fall back to |
||||
|
* guessing the correct value otherwise. |
||||
|
*/ |
||||
|
void init_dpi(void) { |
||||
|
xcb_xrm_database_t *database = NULL; |
||||
|
char *resource = NULL; |
||||
|
|
||||
|
if (conn == NULL) { |
||||
|
goto init_dpi_end; |
||||
|
} |
||||
|
|
||||
|
database = xcb_xrm_database_from_default(conn); |
||||
|
if (database == NULL) { |
||||
|
DEBUG("Failed to open the resource database.\n"); |
||||
|
goto init_dpi_end; |
||||
|
} |
||||
|
|
||||
|
xcb_xrm_resource_get_string(database, "Xft.dpi", NULL, &resource); |
||||
|
if (resource == NULL) { |
||||
|
DEBUG("Resource Xft.dpi not specified, skipping.\n"); |
||||
|
goto init_dpi_end; |
||||
|
} |
||||
|
|
||||
|
char *endptr; |
||||
|
double in_dpi = strtod(resource, &endptr); |
||||
|
if (in_dpi == HUGE_VAL || dpi < 0 || *endptr != '\0' || endptr == resource) { |
||||
|
DEBUG("Xft.dpi = %s is an invalid number and couldn't be parsed.\n", resource); |
||||
|
dpi = 0; |
||||
|
goto init_dpi_end; |
||||
|
} |
||||
|
dpi = (long)round(in_dpi); |
||||
|
|
||||
|
DEBUG("Found Xft.dpi = %ld.\n", dpi); |
||||
|
|
||||
|
init_dpi_end: |
||||
|
if (resource != NULL) { |
||||
|
free(resource); |
||||
|
} |
||||
|
|
||||
|
if (database != NULL) { |
||||
|
xcb_xrm_database_free(database); |
||||
|
} |
||||
|
|
||||
|
if (dpi == 0) { |
||||
|
DEBUG("Using fallback for calculating DPI.\n"); |
||||
|
dpi = init_dpi_fallback(); |
||||
|
DEBUG("Using dpi = %ld\n", dpi); |
||||
|
} |
||||
|
} |
||||
|
|
||||
|
/*
|
||||
|
* This function returns the value of the DPI setting. |
||||
|
* |
||||
|
*/ |
||||
|
long get_dpi_value(void) { |
||||
|
return dpi; |
||||
|
} |
||||
|
|
||||
|
/*
|
||||
|
* Convert a logical amount of pixels (e.g. 2 pixels on a “standard” 96 DPI |
||||
|
* screen) to a corresponding amount of physical pixels on a standard or retina |
||||
|
* screen, e.g. 5 pixels on a 227 DPI MacBook Pro 13" Retina screen. |
||||
|
* |
||||
|
*/ |
||||
|
int logical_px(const int logical) { |
||||
|
if (screen == NULL) { |
||||
|
/* Dpi info may not be available when parsing a config without an X
|
||||
|
* server, such as for config file validation. */ |
||||
|
return logical; |
||||
|
} |
||||
|
|
||||
|
/* There are many misconfigurations out there, i.e. systems with screens
|
||||
|
* whose dpi is in fact higher than 96 dpi, but not significantly higher, |
||||
|
* so software was never adapted. We could tell people to reconfigure their |
||||
|
* systems to 96 dpi in order to get the behavior they expect/are used to, |
||||
|
* but since we can easily detect this case in code, let’s do it for them. |
||||
|
*/ |
||||
|
if ((dpi / 96.0) < 1.25) |
||||
|
return logical; |
||||
|
return ceil((dpi / 96.0) * logical); |
||||
|
} |
@ -0,0 +1,22 @@ |
|||||
|
#pragma once |
||||
|
|
||||
|
/**
|
||||
|
* Initialize the DPI setting. |
||||
|
* This will use the 'Xft.dpi' X resource if available and fall back to |
||||
|
* guessing the correct value otherwise. |
||||
|
*/ |
||||
|
void init_dpi(void); |
||||
|
|
||||
|
/**
|
||||
|
* This function returns the value of the DPI setting. |
||||
|
* |
||||
|
*/ |
||||
|
long get_dpi_value(void); |
||||
|
|
||||
|
/**
|
||||
|
* Convert a logical amount of pixels (e.g. 2 pixels on a “standard” 96 DPI |
||||
|
* screen) to a corresponding amount of physical pixels on a standard or retina |
||||
|
* screen, e.g. 5 pixels on a 227 DPI MacBook Pro 13" Retina screen. |
||||
|
* |
||||
|
*/ |
||||
|
int logical_px(const int logical); |
@ -0,0 +1,25 @@ |
|||||
|
# vim:ft=Dockerfile |
||||
|
FROM debian:sid |
||||
|
|
||||
|
RUN echo force-unsafe-io > /etc/dpkg/dpkg.cfg.d/docker-apt-speedup |
||||
|
# Paper over occasional network flakiness of some mirrors. |
||||
|
RUN echo 'APT::Acquire::Retries "5";' > /etc/apt/apt.conf.d/80retry |
||||
|
|
||||
|
# NOTE: I tried exclusively using gce_debian_mirror.storage.googleapis.com |
||||
|
# instead of httpredir.debian.org, but the results (Fetched 123 MB in 36s (3357 |
||||
|
# kB/s)) are not any better than httpredir.debian.org (Fetched 123 MB in 34s |
||||
|
# (3608 kB/s)). Hence, let’s stick with httpredir.debian.org (default) for now. |
||||
|
|
||||
|
# Install mk-build-deps (for installing the i3 build dependencies), |
||||
|
# clang and clang-format-3.8 (for checking formatting and building with clang), |
||||
|
# lintian (for checking spelling errors), |
||||
|
# test suite dependencies (for running tests) |
||||
|
RUN apt-get update && \ |
||||
|
DEBIAN_FRONTEND=noninteractive apt-get install -y --no-install-recommends \ |
||||
|
build-essential clang git autoconf automake libxcb-randr0-dev pkg-config libpam0g-dev \ |
||||
|
libcairo2-dev libxcb1-dev libxcb-dpms0-dev libxcb-image0-dev libxcb-util0-dev \ |
||||
|
libxcb-xrm-dev libev-dev libxcb-xinerama0-dev libxcb-xkb-dev libxkbcommon-dev \ |
||||
|
libxkbcommon-x11-dev && \ |
||||
|
rm -rf /var/lib/apt/lists/* |
||||
|
|
||||
|
WORKDIR /usr/src |
Loading…
Reference in new issue